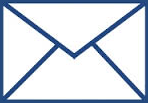
Description
Google Chrome is a cross-platform web browser developed by Google. It was first released in 2008 for Microsoft Windows, and was later ported to Linux, macOS, iOS, and Android. The browser is also the main component of Chrome OS, where it serves as the platform for web apps. more…
Download
New Chrome browser is available here:
https://enterprise.google.com/intl/en_version/chrome/chrome-browser/ mirror
Size
55.5 MB
Silent Install
setup.msi /quiet /norestart
Install Location (10 Folders, 99 Files, 431 MB)
C:\Program Files (x86)\Google\Chrome\Application\76.0.3809.132
Silent Uninstall
msiexec /x{B5FD80C4-8DA4-3815-958F-D6E4AFB1C5D0} /qn /norestart
“C:\Program Files (x86)\Google\Chrome\Application\76.0.3809.132\Installer\setup.exe” –uninstall –multi-install –chrome –system-level –force-uninstall
Registry
HKEY_LOCAL_MACHINE\SOFTWARE\Microsoft\Windows\CurrentVersion\Uninstall\{B5FD80C4-8DA4-3815-958F-D6E4AFB1C5D0}

App GUID
{B5FD80C4-8DA4-3815-958F-D6E4AFB1C5D0}
MSI Property Table
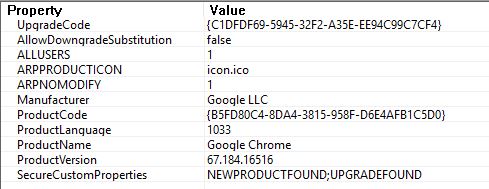
Notes
Download Chrome for Mac
Release Notes v76: July 30, 2019
Chrome Platform Status
Chrome v76 Features
Admin Insider: What’s new in Chrome Enterprise, Release v76
Chrome Scrubber
Mac – Disable Chrome Auto Updates
Method 1
On Mac, you can go to “Users > Your Mac Drive > Library > Google > GoogleSoftwareUpdate” and rename this folder.
Method 2
Open Finder and go to “Applications” folder.
Right click or control + click on the Google Chrome folder and go to “Show Packaged Content”.
Click “Contents” folder and open “Info.plist” file. Remember you need to have editors like Xcode to open plist file. Also you should have write permission for both “Contents” folder and “Info.plist” file to edit.
Look for “KSUpdateURL” key. In our case this is pointing to “https://tools.google.com/service/update2”.
Simply rename the file to something else and save your changes.
Method 3
#!/bin/sh
Version=$(/Applications/Google\ Chrome.app/Contents/MacOS/Google\ Chrome --version | awk '{print $3}')
sudo rm -rf /Applications/Google\ Chrome.app/Contents/Frameworks/Google\ Chrome\ Framework.framework/Versions/"$Version"/Frameworks/KeystoneRegistration.framework
tags: MrNetTek