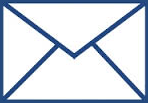
creating a scripted package for Macs…
Initiating and completing the SCCM Agent install and Mac PKI enrollment process is nowhere as easy as it should be. Microsoft has went out of its way to force you to manually enter a password for PKI enrollment (older installs, it wasn’t necessary); not great if you’re trying to automate the installation.
I have the whole process working. I thought I would share my scripts and notes here. Maybe they will be helpful; maybe you can even improve the process.
#1 For the Mac SCCM Agent, download the CM DMG from MS, and extract the contents to a folder on a Mac (we’ll be using the contents of that folder to create a single package for deployment).
#2 Make changes and save this script as sccm_enrollment.sh into the folder you created.
#!/bin/bash
## Eddie Jackson
## 05-31-2018
## Version 1.0
##
## Script to install SCCM Agent and launch enrollment script
Clear
## set current directory
DIR=$( cd "$( dirname "${BASH_SOURCE[]}" )" && pwd)
## change directory
cd ${DIR}
## ADD ROOT CERT -- not really necessary if the DMZ is set up properly
## security add-trusted-cert -d -r trustRoot -k /Library/Keychains/System.keychain root-CA.cer
## INSTALL SCCM AGENT
sudo ./ccmsetup
## PKI SERVER
server_address="YourDMZ.Domain.com"
## PKI ACCOUNT NAME
sccmusername=Domain\\UserName
## Now hand off to the expect script to perform the enrollment
sudo ./expect_enrollment.sh $server_address $sccmusername
## DIALOG BOX FUNCTION
function msgBox() {
osascript << EOT
tell app "System Events"
display dialog "$1" buttons {"OK"} default button 1 with icon 1 with title "Software Updates"
## icon 0=stop,icon 1=software,icon 2=caution
return -- Suppress result
end tell
EOT
}
msgBox "Software has been installed. Please restart your computer!"
exit 0
#3 Make changes and save this script as expect_enrollment.sh into the same folder.
#!/usr/bin/expect
## Eddie Jackson
## 05-31-2018
## Version 1.0
## IMPORT VARIABLES FROM THE SCCM SCRIPT
set server_address [lindex $argv 0]; # Get the Server Address from the sccm_enrollment script
set sccmusername [lindex $argv 1]; # Get the sccmuser name form the sccm_enrollment script
# SET TIMEOUT
set timeout 20
## RUN SCRIPT
spawn ./Tools/CMEnroll -s $server_address -ignorecertchainvalidation -u $sccmusername
expect "Please enter your password."
# remember, a complex password may require the backward slash as an escape character
# you could pass this from the sccm script, but with special characters, it fails often
# PASSWORD HERE
send X\(IcAnDoIT\!
send \n
expect "Successfully enrolled"
interact
unset sccmusername
unset server_address
exit 0
#4 If your company requires package branding and/or logging, make sure those are completed before continuing.
#5 Now, using Packages from WhiteBox, create a Distribution package.
#6 Add the extracted files and folders from the SCCM Agent DMG, and the two scripts above, into the Additional Resources under the Scripts tab.
#7 Set the sccm_enrollment.sh script as the Pre-installation script.
#8 Build and Run.
#9 If that works, deploy that package from desktop management software. Note, when deploying from management software, you should not see any Mac GUI setup.
Notes
Guidelines for Mac software packaging