clear-host $string1 = '<a href="https://eddiejackson.net/this_is_a_test.jpg" rel="lightbox noopener" target="_blank"><img class="TEST" src="https://eddiejackson.net/this_is_a_test.jpg" width="264" height="198"></a>' $string2 = '<a href="https://eddiejackson.net/this_is_a_test.jpg" rel="lightbox noopener" target="_blank"><strong></strong></a> <a href="https://eddiejackson.net/this_is_a_test.jpg" rel="lightbox noopener" target="_blank">This is a test </a><a href="https://eddiejackson.net/this_is_a_test.jpg" rel="lightbox noopener" target="_blank"><strong></strong></a>' $string3 = '<a href="https://eddiejackson.net/this_is_a_test.jpg" rel="lightbox noopener" target="_blank"><img class="TEST" src="https://eddiejackson.net/this_is_a_test.jpg" width="264" height="198"></a>' $string4 = '<a href="https://eddiejackson.net/this_is_a_test.jpg" rel="lightbox noopener" target="_blank"><strong></strong></a> <a href="https://eddiejackson.net/this_is_a_test.jpg" rel="lightbox noopener" target="_blank">This is a test </a><a href="https://eddiejackson.net/this_is_a_test.jpg" rel="lightbox noopener" target="_blank"><strong></strong></a>' write-host "{{{ REMOVE HYPERLINKS FROM STRING }}}" write-host "" write-host "" # NOT A BEST USE OF REGEX write-host "INPUT1" $string1 $a = $string1 -replace "<([^>]*)(?:a href|:\w+)=(?:'[^']*'|""[^""]*""|[^\s>]+)([^>]*)>","`$1`$2" # using the -replace - not optimal $b = $a -replace "","" $c = $b -replace 'rel="lightbox"',"" $d = $c -replace 'rel="noopener noreferrer"',"" $e = $d -replace 'target="_blank"',"" $f = $e -replace 'target="_parent"',"" $g = $f -replace 'target="_top"',"" $h = $g -replace 'target="_new"',"" $i = $h -replace 'rel="noopener noreferrer"',"" write-host "" Write-host "OUTPUT1: RETURNS JUST IMG SRC" $i write-host "`n`n" # GETTING WARMER WITH THESE Write-host "INPUT2" $string2 write-host "" Write-host "OUTPUT2: RETURNS EVERYTHING BUT URL" $x = $string2 -replace "<([^>]*)(?:a href|:\w+)=(?:'[^']*'|""[^""]*""|[^\s>]+)([^>]*)>","" $y = $x -replace "",'' $y write-host "`n`n" Write-host "INPUT3" $string3 write-host "" Write-host "OUTPUT3: RETURNS EVERYTHING BUT URL" $x = $string4 -replace ("<([^>]*)(?:a href|:\w+)=(?:'[^']*'|""[^""]*""|[^\s>]+)([^>]*)>",""); $y = $x -replace "","" $y write-host "`n`n" # THIS IS THE BEST USAGE OF REGEX Write-host "INPUT4" $string4 write-host "" Write-host "OUTPUT4: RETURNS EVERYTHING BUT URL" $x = $string4 -replace "<([^>]*)(?:a href|:\w+)=(?:'[^']*'|""[^""]*""|[^\s>]+)([^>]*)>|(","" $x write-host "`n`n"
Screenshot
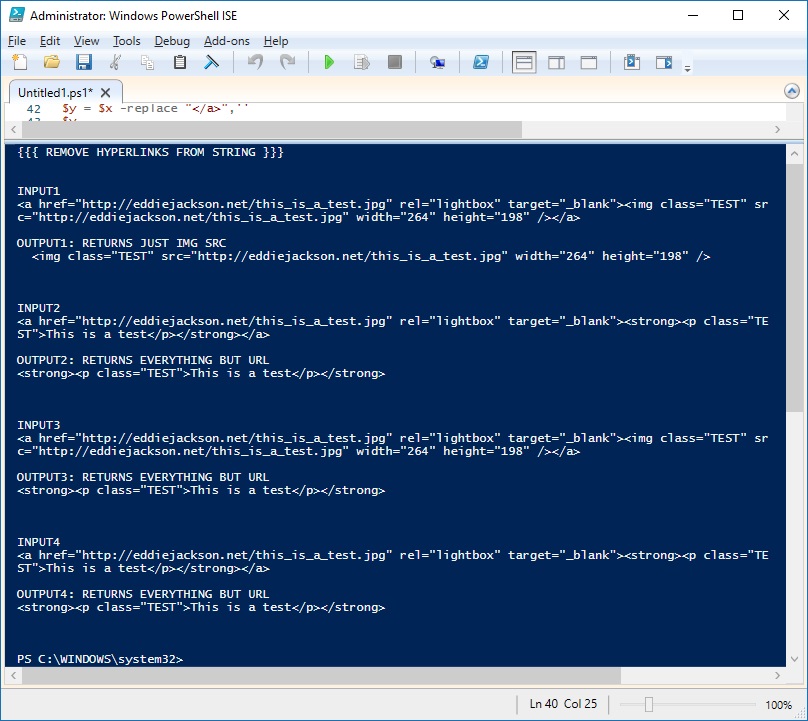