Setting Dynamic Sound Settings on the HP 9470 Laptop.
This changes/updates the sound settings for the HP Laptop that uses random GUIDs in the registry.
Const HKEY_CLASSES_ROOT = &H80000000
Const HKEY_CURRENT_USER = &H80000001
Const HKEY_LOCAL_MACHINE = &H80000002
Const HKEY_USERS = &H80000003
Const REG_SZ = 1
Const REG_EXPAND_SZ = 2
Const REG_BINARY = 3
Const REG_DWORD = 4
Const REG_MULTI_SZ = 7
strComputer = “.”
hDefKey = HKEY_LOCAL_MACHINE
Set oReg = GetObject(“winmgmts:{impersonationLevel=impersonate}!\\” & strComputer & “\root\default:StdRegProv”)
‘THIS CHANGES THE FIRST SET OF KEYS
strKeyPath = “SOFTWARE\IDT\Apo\LFX\MicIn4\Presets\_Initial\SpeexNS”
oReg.EnumKey hDefKey, strKeyPath, arrSubKeys
on error resume next
For Each strSubkey In arrSubKeys
strSubKeyPath = strKeyPath & “\” & strSubkey
oReg.EnumValues hDefKey, strSubKeyPath, arrValueNames, arrTypes
For i = LBound(arrValueNames) To UBound(arrValueNames)
strValueName = arrValueNames(i)
Select Case arrTypes(i)
Case REG_DWORD
oReg.GetDWORDValue hDefKey, strSubKeyPath, strValueName, uValue
‘wscript.echo ” ” & strValueName & ” (REG_DWORD) = ” & CStr(uValue)
‘wscript.echo uValue
if uValue = 0 then
newValue = 1
oReg.SetDWORDValue hDefKey, strSubKeyPath, strValueName, newValue
end if
End Select
Next
Next
‘THIS CHANGES THE SECOND SET OF KEYS
strKeyPath = “SOFTWARE\Microsoft\Windows\CurrentVersion\MMDevices\Audio\Render”
Dim strGUID
oReg.EnumKey hDefKey, strKeyPath, arrSubKeys
on error resume next
For Each strSubkey In arrSubKeys
strSubKeyPath = strKeyPath & “\” & strSubkey & “\” & “Properties”
oReg.EnumValues hDefKey, strSubKeyPath, arrValueNames, arrTypes
For i = LBound(arrValueNames) To UBound(arrValueNames)
strValueName = arrValueNames(i)
Select Case arrTypes(i)
Case REG_SZ
oReg.GetStringValue hDefKey, strSubKeyPath, strValueName, strValue
‘wscript.echo ” ” & strValueName & ” (REG_SZ) = ” & strValue
if strValue = “Speakers / HP” then
strGUID = strSubKeyPath
end if
End Select
Next
Next
strKeyPath = strGUID
oReg.EnumKey hDefKey, strKeyPath, arrSubKeys
on error resume next
For Each strSubkey In arrSubKeys
strSubKeyPath = strKeyPath & “\” & strSubkey
oReg.EnumValues hDefKey, strSubKeyPath, arrValueNames, arrTypes
For i = LBound(arrValueNames) To UBound(arrValueNames)
strValueName = arrValueNames(i)
Select Case arrTypes(i)
Case REG_DWORD
oReg.GetDWORDValue hDefKey, strSubKeyPath, strValueName, uValue
‘wscript.echo ” ” & strValueName & ” (REG_DWORD) = ” & CStr(uValue)
if uValue = 0 then
newValue = 1
oReg.SetDWORDValue hDefKey, strSubKeyPath, strValueName, newValue
end if
End Select
Next
Next
Wscript.quit(0)
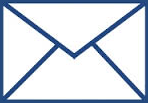