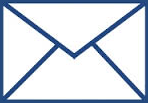
Citrix Workspace app for Windows
Citrix Workspace app for Windows is an easy-to-install software that provides access to your applications and desktops using XenApp and XenDesktop from a remote client device. Citrix Workspace app provides access from your desktop, Start menu, Citrix Workspace user interface, or web browsers.
You can use Citrix Workspace app on domain and non-domain joined PCs, tablets, and thin clients. Using Citrix StoreFront in conjunction with Citrix Workspace app allows your organization to provide you with self-service access to your applications and desktops – all with a common user interface, regardless of the endpoint device hardware, operating system (32-bit and 64-bit editions), or form factor.
Silent Command
citrix.exe” /rcu /silent STORE0=”Store;https://mycitrix.umc.edu/Citrix/ummcWeb/discovery;Store”
Downloads
CitrixWorkspace_ADMX_Files.zip
CitrixWorkspace_Installation_Scripts.zip
CitrixCtxReceiverCmdLineHelper.zip
more…
About Citrix Workspace app
When powered by Citrix Workspace services, the new Citrix Workspace app gives users instant access to all their SaaS and web apps, their files and mobile apps, and their virtual apps and desktops from an easy-to-use, all-in-one interface. Citrix Workspace app is a single point of entry to all workspace services for users. Users get seamless and secure access to all the apps they need to stay productive, including features such as embedded browsing and single sign-on.
Citrix Workspace app aggregates and incorporates the full capabilities of Citrix Receiver as well as other Citrix client technologies – including HDX, the NetScaler plug-ins, and Citrix Endpoint Management Secure Hub. Citrix Workspace app also has full integration of Citrix Content Collaboration (formerly ShareFile) in the new Files tab. With this integration, all your data is accessible within Citrix Workspace app. The ability to upload and download files and even open files within Citrix Workspace app are now integrated into one application. Citrix Workspace app is also enhanced to deliver additional capabilities regarding data loss prevention, secure access to SaaS apps, secure internet browsing capabilities, advanced search, and more.
Workspace configuration
Citrix Workspace app for Windows supports configuring Workspaces for subscribers, who might be using one or more services available from Citrix Cloud.
Citrix Workspace app will intelligently display only the specific workspace resources to which users are entitled. All your digital workspace resources available in Citrix Workspace app are powered by the Citrix Cloud Workspace experience service.
A workspace is part of a digital workspace solution that enables IT to securely deliver access to apps from any device. This screenshot is an example of what the workspace experience looks like to your subscribers. This interface is evolving and may look different to what your subscribers are working with today. For example, it might say “StoreFront” at the top of the page instead of “Workspace”.
Scripts for Deploying Citrix Workspace app for Windows
This ZIP file contains sample scripts to deploy and configure Citrix Workspace app. It is an optional download, provided on an as-is basis by Citrix to serve as an example. Before use, IT administrators must customize the scripts to suit their environment. The uninstall and install scripts may be used as noted in the upgrade guide for Citrix Workspace app for Windows (CTX135933). Version: 18.9.0.19526 (1809)
Citrix ADMX/ ADML Templates for Group Policy Editor
This ZIP file contains ADMX and ADML files to deploy and configure Citrix Workspace app using group policy object editor. It is an optional download, to be used by IT administrators, and not meant for use by end-users. The administrative templates (ADMX and ADML files) may be used as noted in the upgrade guide for Citrix Workspace app for Windows (CTX135933). Version: 18.9.0.19526 (1809)
Citrix Receiver CmdLine Tool 1.0

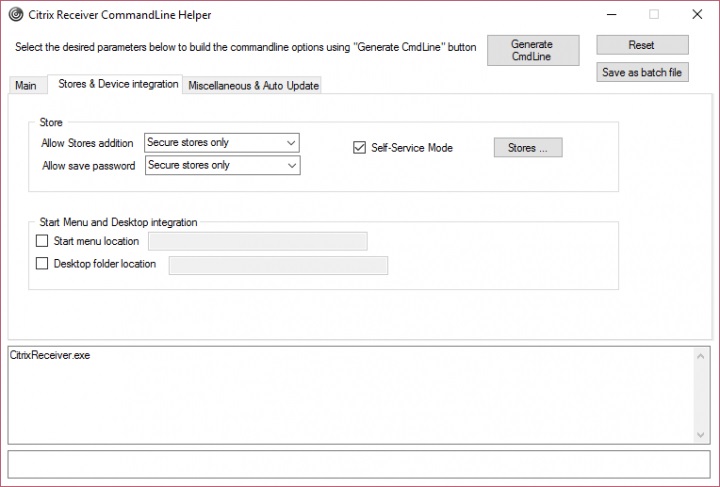
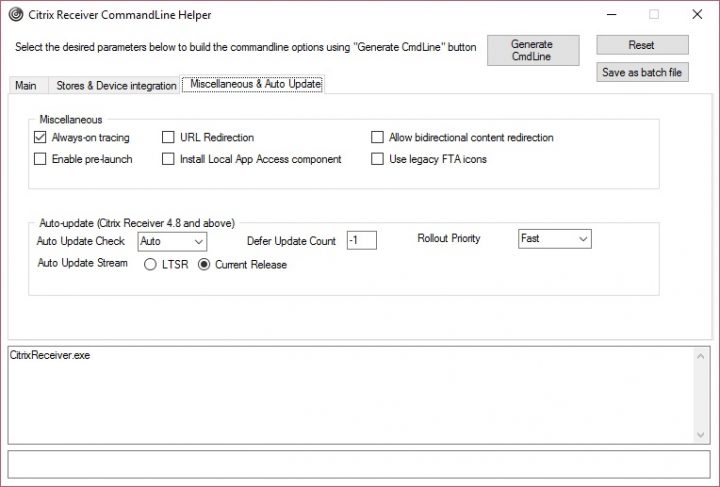
Select the options in the GUI, click on “Generate CmdLine” and the tool will display the full commandline in the tool, as well as copying it to the clipboard.
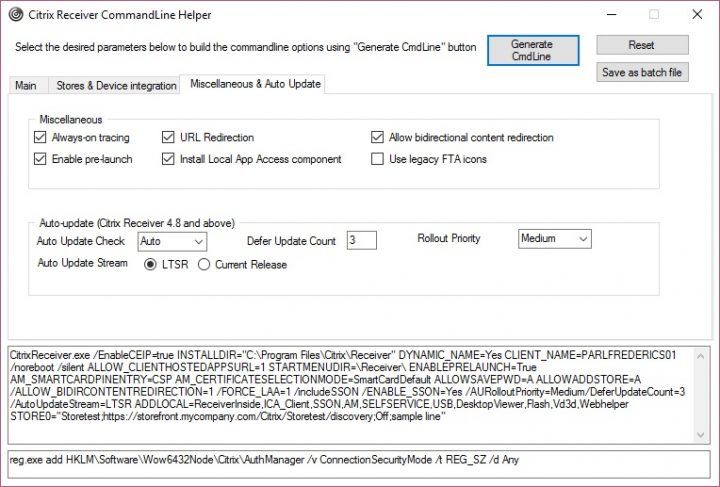
When clicking on “Save as batch file,” the tool will generate a batch file ready to use (including the registry entry if needed)
Notes
Configure and install using command-line parameters
August 6, 2018
Customize Citrix Receiver for Windows installer by specifying command line options. The installer package self-extracts to the user’s temp directory before launching the setup program. The space requirement includes program files, user data, and temp directories after launching several applications.
For more information about system requirements, see System requirements.
To install Citrix Receiver for Windows from a command prompt, use the syntax:
CitrixReceiver.exe [Options]
Receiver Updates
Option |
/AutoUpdateCheck = auto/manual/disabled |
Description |
Indicates that Citrix Receiver for Windows detects when an update is available. Auto – You are notified when an update is available (default).; Manual –You are not notified when updates are available. Check for updates manually.; Disabled – Disable auto-update |
Sample usage |
CitrixReceiver.exe /AutoUpdateCheck = auto ; CitrixReceiver.exe /AutoUpdateCheck = manual ; CitrixReceiver.exe /AutoUpdateCheck = disabled |
Option |
/AutoUpdateStream= LTSR/Current |
Description |
Indicates the release of Citrix Receiver for Windows. LTSR – indicates that the release is a Long Term Service Release ; Current – indicates that the release is the latest version of Citrix Receiver for Windows |
Sample usage |
CitrixReceiver.exe /AutoUpdateStream= LTSR ; CitrixReceiver.exe /AutoUpdateStream= Current |
Option |
/DeferUpdateCount |
Description |
Indicates the release of Citrix Receiver for Windows. -1 – indicates that you can defer the notifications any number of times (default value=-1) ; 0 – indicates that the Remind me later option is not displayed. Any other number – indicates that the Remind me later option is displayed in that count. For example, if you set the value to 10, the Remind me later option is displayed 10 times. |
Sample usage |
CitrixReceiver.exe /DeferUpdateCount=-1 ; CitrixReceiver.exe /DeferUpdateCount=-0 ; CitrixReceiver.exe /DeferUpdateCount=any other number |
Option |
/AURolloutPriority |
Description |
Indicates the period when you can stage the rollout. Fast – Update rollout happens at the beginning of the delivery period. Medium – Update rollout happens at the mid-delivery period. Slow – Update rollout happens at the end of the delivery period. |
Sample usage |
CitrixReceiver.exe /AURolloutPriority=Fast ; CitrixReceiver.exe /AURolloutPriority=Medium ; CitrixReceiver.exe /AURolloutPriority=Slow |
Enable bidirectional content redirection
Note
By default, Citrix Receiver for Windows does not install the bidirectional content redirection components if they are already installed on the server. If you are using XenDesktop as a client machine, you must install Citrix Receiver for Windows by using the /FORCE_LAA switch to install the bidirectional content redirection components. The feature, however, must be configured both on the server and the client.
Option |
ALLOW_BIDIRCONTENTREDIRECTION=1 |
Description |
Indicates that the bidirectional content redirection between client to host and host to the client is Enabled. |
Sample usage |
CitrixReceiver.exe /ALLOW_BIDIRCONTENTREDIRECTION=1 |
Hide Settings Option
|
|
Option |
/DisableSetting |
Description |
Suppresses Settings Option to be displayed in the Advanced Preferences dialog. |
Sample usage |
CitrixReceiver.exe /DisableSetting=3 |
|
|
If you want both Application Display and Reconnect Options to be displayed in the Settings Option.. |
Enter CitrixReceiver.exe /DisableSetting=0 |
If you want Settings Option to be hidden in the Advanced Preferences dialog |
Enter CitrixReceiver.exe /DisableSetting=3 |
If you want Settings Option to display only Application Display |
Enter CitrixReceiver.exe /DisableSetting=2 |
If you want Settings Option to display only Reconnect Options |
Enter CitrixReceiver.exe /DisableSetting=1 |
Enable Local App Access
Option |
FORCE_LAA=1 |
Description |
By default, Citrix Receiver for Windows does not install the client side Local App Access components if the components are already installed on the server. To force the client side Local App Access components on the Citrix Receiver, use FORCE_LAA command line switch. Administrator-level privileges are required to perform these steps. For more information on Local App Access, see Local App Access in XenApp and XenDesktop documentation. |
Sample usage |
CitrixReceiver.exe /FORCE_LAA =1 |
Display usage information
Option |
/? or /help |
Description |
Indicates usage information |
Sample usage |
CitrixReceiver.exe /? ; CitrixReceiver.exe /help |
Suppress reboot during UI installation
Option |
/noreboot |
Description |
Suppresses reboot during UI installations. This option is not necessary for silent installs. If you suppress reboot prompts, the USB devices that are in suspended state when Citrix Receiver for Windows installs is not recognized by Citrix Receiver for Windows until after the user device is restarted. |
Sample usage |
CitrixReceiver.exe /noreboot |
Silent installation
Option |
/silent |
Description |
SDisables the error and progress dialogs to run a completely silent installation. |
Sample usage |
CitrixReceiver.exe /silent |
Enable single sign on authentication
Option |
/includeSSON |
Description |
Indicates that the Citrix Receiver for Windows will be installed with the single sign-on component. The related option, ENABLE_SSON, is enabled when /includeSSON is on the command line. If you use ADDLOCAL= to specify features and you want to install the single sign on, you must also specify the value SSON. To enable pass-through authentication for a user device, you must install Citrix Receiver for Windows with local administrator rights from a command line that has the option /includeSSON. For more information, see How to Manually Install and Configure Citrix Receiver for Pass-Through Authentication. Note: Smart card, Kerberos and Local user name and password policies are inter-dependent. The order of configuration is important. We recommend to first disable unwanted policies, and then enable the policies you require. Carefully validate the result. |
Sample usage |
CitrixReceiver.exe /includeSSON |
Enable single sign on when /includeSSON is specified
Option |
ENABLE_SSON={Yes, No} |
Description |
Enable Single sign-on when /includeSSON is specified. The default value is Yes. This property is required for smart card Single sign-on. Users must log off and log in to their devices after installing with Single sign-on authentication enabled. Requires administrator rights. |
Sample usage |
CitrixReceiver.exe ENABLE_SSON=Yes |
Always-on tracing
Option |
/EnableTracing={true, false} |
Description |
By default, this feature is set to true. Use this property to enable or disable the always-on tracing feature. Always-on tracing helps to collect critical logs around the connection time. These logs can prove useful when troubleshooting intermittent connectivity issues. The Always On tracing policy overrides this setting. |
Sample usage |
CitrixReceiver.exe /EnableTracing=true |
Using the Citrix Customer Experience Improvement Program (CEIP)
Option |
EnableCEIP={true , false } |
Description |
When you enable participation in the Citrix Customer Experience Improvement Program (CEIP), anonymous statistics and usage information are sent to Citrix to help Citrix improve the quality and performance of its products. |
Sample usage |
CitrixReceiver.exe EnableCEIP=true |
Specify the installation directory
Option |
INSTALLDIR=Installation Directory |
Description |
Specifies the installation path where most of the Citrix Receiver software is installed. The default value is C:\Program Files\Citrix\Receiver. The following Receiver components are installed in the C:\Program Files\Citrix path: Authentication Manager ; Citrix Receiver ; Self-Service plug-in. If you use this option and specify an Installation directory, you must install RIInstaller.msi file in the \Receiver directory and the other .msi files in the installation directory. |
Sample usage |
CitrixReceiver.exe INSTALLDIR=c:\Citrix\Test |
Identify a user device
Option |
CLIENT_NAME=ClientName |
Description |
Specifies the client name, where ClientName is the name used to identify the user device to the server. The default value is %COMPUTERNAME% |
Sample usage |
CitrixReceiver.exe CLIENT_NAME=%COMPUTERNAME%. |
Dynamic client name
Option |
ENABLE_CLIENT_NAME=Yes, No |
Description |
The dynamic client name feature allows the client name to be the same as the computer name. When users change their computer name, the client name changes to match. Defaults to Yes. To disable dynamic client name support, set this property to No and specify a value for the CLIENT_NAME property. |
Sample usage |
CitrixReceiver.exe ENABLE_DYNAMIC_CLIENT_NAME =Yes |
Install specified components
Option |
ADDLOCAL=<feature… ,> |
Description |
Installs set of local features specified in ADDLOCAL. |
Sample usage |
CitrixReceiver.exe ADDLOCAL=ReceiverInside,ICA_Client,AM,SELFSERVICE,DesktopViewer,Flash,Vd3d,usb,WebHelper |
Configure Citrix Receiver for Windows to manually add Stores
Option |
ENABLE_CLIENT_NAME=Yes, No |
Description |
The dynamic client name feature allows the client name to be the same as the computer name. When users change their computer name, the client name changes to match. Defaults to Yes. To disable dynamic client name support, set this property to No and specify a value for the CLIENT_NAME property. |
Sample usage |
CitrixReceiver.exe ENABLE_DYNAMIC_CLIENT_NAME =Yes |
Save credentials for stores locally using PNAgent protocol
Option |
ALLOWSAVEPWD={N, S, A} |
Description |
The default is the value specified by the PNAgent server at runtime. Specifies whether users can save the credentials for stores locally on their computers and apply only to stores using the PNAgent protocol. Defaults to S. Options include: N – Never allow users to save their passwords. S – Allow users to save passwords for both secure stores (HTTPS) and non-secure stores (HTTPS) and non-secure stores (HTTP). You can also control this feature by updating the registry key HKLM\Software[Wow6432Node]\Citrix\Dazzle\AllowSavePwd. Note: The following registry key must be added manually if AllowSavePwd does not work: 1 Key for 32 bit OS client: HKLM\Software\Citrix\AuthManager 2 Key for 64 bit OS client: HKLM\Software\wow6432node\Citrix\AuthManager 3 Type: REG_SZ 4 Value: never – never allow users to save their passwords. secureonly – allow users to save passwords for secure stores only (configured with HTTPS). always – allow users to save passwords for both secure stores (HTTPS) and non-secure stores (HTTP). |
Sample usage |
CitrixReceiver.exe ALLOWADDSTORE=N |
Select certificate
Option |
AM_CERTIFICATESELECTIONMODE={Prompt, SmartCardDefault, LatestExpriry } |
|
|
Description |
Use this option to select a certificate. The default value is Prompt, which prompts the user to choose a certificate from a list. Change this property to choose the default certificate (per the smart card provider) or the certificate with the latest expiry date. If there are no valid logon certificates, the user is notified, and given the option to use an alternate logon method if available.You can also control this feature by updating the registry key HKCU or HKLM\Software[Wow6432Node]Citrix\AuthManager:CertificateSelectionMode={ Prompt |
SmartCardDefault |
LatestExpiry }. Values defined in HKCU take precedence over values in HKLM to best assist the user in selecting a certificate. |
Sample usage |
CitrixReceiver.exe AM_CERTIFICATESELECTIONMODE=Prompt |
|
|
Use CSP components to manage Smart Card PIN entry
Option |
AM_SMARTCARDPINENTRY=CSP |
Description |
Use CSP components to manage Smart Card PIN entry. By default, the PIN prompts presented to users are provided by Citrix Receiver rather than the smart card Cryptographic Service Provider (CSP). Receiver prompts users to type a PIN when required and then passes the PIN to the smart card CSP. Specify this property to use the CSP components to manage the PIN entry, including the prompt for a PIN. |
Sample usage |
CitrixReceiver.exe AM_SMARTCARDPINENTRY=CSP |
Using Kerberos
Option |
ENABLE_KERBEROS={Yes , No} |
Description |
The default value is No. Specifies whether the HDX engine should use Kerberos authentication and applies only when single sign-on (pass-through) authentication is enabled. For more information, see Configure domain pass-through authentication with Kerberos. |
Sample usage |
CitrixReceiver.exe ENABLE_KERBEROS=No |
Displaying legacy FTA icons
Option |
LEGACYFTAICONS={False, True} |
Description |
Use this option to display Legacy FTA icons. The default value is False. Specifies whether or not application icons are displayed for documents that have file type associations with subscribed applications. When the argument is set to false, Windows generates icons for documents that do not have a specific icon assigned to them. The icons generated by Windows consist of a generic document icon overlaid with a smaller version of the application icon. Citrix recommends enabling this option if you plan to deliver Microsoft Office applications to users running Windows 7. |
Sample usage |
CitrixReceiver.exe LEGACYFTAICONS=False |
Enabling pre-launch
Option |
ENABLEPRELAUNCH={False, True} |
Description |
The default value is False. For information about session pre-launch, see Reduce application launch time. |
Sample usage |
CitrixReceiver.exe ENABLEPRELAUNCH=False |
Option |
STARTMENUDIR={Directory Name} |
Description |
By default, applications appear under Start > All Programs. You can specify the relative path of the shortcuts in the programs folder. For example, to place shortcuts under Start > All Programs > Receiver, specify STARTMENUDIR=\Receiver. You can change or move the folder at any time.You can also control this feature using a registry key: Create the entry REG_SZ for StartMenuDir and give it the value “\RelativePath” Location: HKEY_LOCAL_MACHINE\Software[Wow6432Node]Citrix\Dazzle , HKEY_CURRENT_USER\Software\Citrix\Dazzle. For applications published through XenApp with a Client applications folder (also referred to as a Program Neighborhood folder) specified, you can specify that the client applications folder is to be appended to the shortcuts path as follows: Create the entry REG_SZ for UseCategoryAsStartMenuPath and give it the value “true.” Use the same registry locations as noted above.Note: Windows 8/8.1 does not allow you to create nested folders within the Start menu. Applications are displayed individually or inside the root folder and not within defined Category sub folders. Examples: 1 If client application folder is \office, UseCategoryAsStartMenuPath is true, and no StartMenuDiris specified, shortcuts are placed under Start > All Programs > Office. 2 If Client applications folder is \Office, UseCategoryAsStartMenuPath is true, and StartMenuDir is \Receiver, shortcuts are placed under Start > All Programs > Receiver > Office. Changes made to these settings have no impact on shortcuts that are already created. To move shortcuts, you must uninstall and reinstall the applications |
Sample usage |
CitrixReceiver.exe STARTMENUDIR=\Office |
Specifying the Store Name
Option |
STOREx=”storename;http[s]://servername.domain/IISLocation/discovery;[On, Off] ; [storedescription] “[STOREy=”–”] |
Description |
Use this option to specify the Store name. Specifies up to 10 stores to use with Citrix Receiver. Values: x and y – Integers 0 through 9.; storename – Defaults to store. This must match the name configured on the StoreFront Server.; servername.domain – The fully qualified domain name of the server hosting the store. ; IISLocation – the path to the store within IIS. The store URL must match the URL in StoreFront provisioning files. The store URLs are of the form “/Citrix/store/discovery.” To obtain the URL, export a provisioning file from StoreFront, open it in notepad and copy the URL from the Address element.; On, Off – The optional Off configuration setting enables you to deliver disabled stores, giving users the choice of whether or not they access them. When the store status is not specified, the default setting is On. storedescription – An optional description of the store, such as HR App Store.Note: In this release, it is important to include “/discovery” in the store URL for successful pass-through authentication. |
Sample usage |
CitrixReceiver.exe STORE0=”Store;https://test.xx.com/Citrix/Store/Discovery” |
Enabling URL Redirection on user devices
Option |
ALLOW_CLIENTHOSTEDAPPSURL=1 |
Description |
Enables the URL redirection feature on user devices. Requires administrator rights. Requires that Citrix Receiver is installed for All Users. For information about URL redirection, see Local App Access and its subtopics in the XenDesktop 7 documentation. |
Sample usage |
CitrixReceiver.exe ALLOW_CLIENTHOSTEDAPPSURL=1 |
Enabling self-service mode
Option |
SELFSERVICEMODE={False , True} |
Description |
The default value is True. When the administrator sets the SelfServiceMode flag to false, the user no longer has access to the self-service Citrix Receiver user interface. Instead, they can access subscribed apps from the Start menu and via desktop shortcuts – known as “shortcut-only mode”. |
Sample usage |
CitrixReceiver.exe SELFSERVICEMODE=False |
Specifying the directory for Desktop Shortcuts
Option |
DESKTOPDIR=Directory Name |
Description |
Brings all shortcuts into a single folder. Category Path is supported for desktop shortcuts. Note: When using the DESKTOPDIR option, set the PutShortcutsOnDesktop key to True. |
Sample usage |
CitrixReceiver.exe DESKTOPDIR=\Office |
Upgrading from an unsupported Citrix Receiver version
Note
When you upgrade Citrix Receiver Version 13.x Enterprise or 12.x to Citrix Receiver for Windows Version 4.4 and later using the graphical user interface, the installer runs the Receiver cleanup utility by default.
However, the utility does not run by default when you upgrade from the command line. To upgrade from the command line, run the following command:
CitrixReceiver.exe /rcu /silent
When you upgrade Citrix Receiver for Windows from 13.x (non-Enterprise) or 4.1 to Version 4.2 or later, the /rcu switch is unnecessary and ignored.
Option |
/rcu |
Description |
Allows you to upgrade from an unsupported version to the latest version of Citrix Receiver. |
Sample usage |
CitrixReceiver.exe /rcu |
Troubleshooting the installation
If there is a problem with the installation, search in the user’s %TEMP%/CTXReceiverInstallLogs directory for the logs with the prefix CtxInstall- or TrolleyExpress- . For example:
CtxInstall-ICAWebWrapper-20141114-134516.log
TrolleyExpress-20090807-123456.log
Examples of a command line installation
To specify the NetScaler Gateway store URL:
CitrixReceiver.exe STORE0=”<StoreFriendlyName>;testserver <StoreName>;<On/Off>;<StoreDescription>”]
Note: The NetScaler Gateway store URL must be the first entry in the list of configured store URLs.
To install all components silently and specify two application stores:

To specify single sign-on (pass-through authentication) and add a store that points to a XenApp Services URL:
